using System;
using System.Data;
using aspNetEmail;
using aspNetPOP3;
using aspNetMime;
using AdvancedIntellect;
using System.Data.SqlClient;
using System.Data.SqlTypes;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Text;
using System.Net.Sockets;
public partial class mailrouting : System.Web.UI.Page
{
POP3 pop;
// POP3ConnectionException ex = new POP3ConnectionException();
string primary, primarypass, mail1, mailpass1, mail2, mailpass2, id, pwd;
int mcount = 0;
SqlConnection con = new SqlConnection("Data Source=.;Initial Catalog=Routingmail;Integrated Security=True");
protected void Page_Load(object sender, EventArgs e)
{
con.Open();
String userid = Session["userid"].ToString();
SqlCommand cmd = new SqlCommand("select * from phoneemail where userid='" + userid + "'", con);
SqlDataReader rd = cmd.ExecuteReader();
if (rd.Read())
{
primary = rd[1].ToString();
primarypass = rd[2].ToString();
mail1 = rd[4].ToString();
mailpass1 = rd[5].ToString();
mail2 = rd[6].ToString();
mailpass2 = rd[7].ToString();
}
rd.Close();
con.Close();
for (int i = 1; i < 4; i++)
{
switch (i)
{
case 1:
id = mail1;
pwd = mailpass1;
break;
case 2:
id = mail2;
pwd = mailpass2;
break;
default:
break;
}
string ab = id.Substring(id.IndexOf('@') + 1);
if (ab.Equals("gmail.com"))
{
pop = new POP3("pop.gmail.com", id, pwd);
AdvancedIntellect.Ssl.SslSocket ss1 = new AdvancedIntellect.Ssl.SslSocket();
pop.LoadSslSocket(ss1);
pop.Port = 995;
}
else
{
pop = new POP3("mail.ideonics.co.in", id, pwd);
}
pop.LogInMemory = true;
pop.Connect();
//pop.Connect(id, pwd, "mail.ideonics.co.in");
pop.PopulateInboxStats();
int count = pop.MessageCount();
if (count != 0)
{
for (int j = 0; j < count; j++)
{
try
{
if (pop.GetMessage(j).Subject != null)
{
if (!pop.GetMessage(j).Subject.Value.Equals("Reply"))
{
MimeMessage m = pop.GetMessage(j);
forward(m);
Reply(m.Subject.Value);
pop.Delete(j);
Label2.Text = "Mails are sending............" + mcount;
}
}
}
catch (Exception ex)
{
Response.Write(ex.Message);
break;
}
}
Label2.Text = "Total Mails sent................." + mcount;
pop.Disconnect();
con.Close();
}
}
}
public void forward(MimeMessage m)
{
mcount++;
StringBuilder inlineEmail = new StringBuilder();
inlineEmail.Append("---------- original Message---------\r\n");
if (m.From != null)
{
inlineEmail.Append("From:" + m.From.ToString() + "\r\n");
}
if ((m.To != null) && (m.To.Count > 0))
{
inlineEmail.Append("to:" + m.To.ToString() + "\r\n");
}
if (m.Date != null)
{
inlineEmail.Append("Date:" + m.Date + "\r\n");
}
if (m.Subject != null)
{
inlineEmail.Append("SUBJECT: " + aspNetMime.Header.DeocodeHeaderValue(m.Subject.Value) + "\r\n");
}
if (m.TextMimePart != null)
{
inlineEmail.Append("\r\n");
inlineEmail.Append(m.TextMimePart.DecodedText());
}
MimePartCollection attachments = m.Attachments;
EmailMessage email = new EmailMessage();
email.Server = "mail.ideonics.co.in";
email.FromAddress = id;
email.To = primary;
email.Subject = "Mail from" + id;
email.Body = "see email below\r\n\r\n";
email.Body += inlineEmail.ToString();
if ((attachments != null) && (attachments.Count > 0))
{
for (int i = 0; i < attachments.Count; i++)
{
//add each attachment to the email message
Attachment a = new Attachment(attachments[i].Data(), attachments[i].AttachmentName());
email.AddAttachment(a);
}
}
email.Send();
}
public void Reply(string sub)
{
EmailMessage email = new EmailMessage();
email.Server = "mail.ideonics.co.in";
email.FromAddress = primary;
email.To = id;
email.Subject = "Reply";
email.Body = sub + "is forwardedto your primary id" + primary;
email.Send();
}
}
ASP.NET is a development framework for building web pages and web sites with HTML, CSS, JavaScript and server scripting. ASP.NET supports three different development models: Web Pages, MVC (Model View Controller), and Web Forms.
Subscribe to:
Post Comments (Atom)
Using Authorization with Swagger in ASP.NET Core
Create Solution like below LoginModel.cs using System.ComponentModel.DataAnnotations; namespace UsingAuthorizationWithSwagger.Models { ...
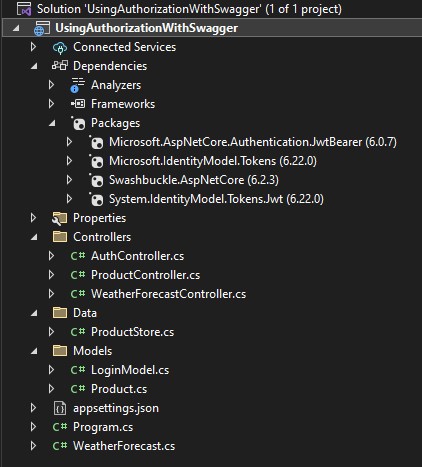
-
<ControlTemplate x:Key="TextBoxBaseControlTemplate" TargetType="{x:Type TextBoxBase}"> <Borde...
-
<?xml version="1.0" encoding="utf-8"?> <Report xmlns:rd="http://schemas.microsoft.com/SQLServer/reporting...
-
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.D...
No comments:
Post a Comment