A simple Program to view xml in Datagrid
using System.Xml;
XmlTextReader reader = new XmlTextReader("http://msdn.microsoft.com/rss.xml");
// creates a new instance of DataSet
DataSet ds = new DataSet();
// Reads the xml into the dataset
ds.ReadXml(reader);
// Assigns the data table to the datagrid
myDataGrid.DataSource = ds.Tables[2];
// Binds the datagrid
myDataGrid.DataBind();
ASP.NET is a development framework for building web pages and web sites with HTML, CSS, JavaScript and server scripting. ASP.NET supports three different development models: Web Pages, MVC (Model View Controller), and Web Forms.
Monday, April 28, 2008
Using RSS Feeds with Asp.net
RSS stands for (Really Simple Syndication). Basically RSS feeds are xml files which are provided by many websites so you can view their contents on your own websites rather than browsing their site. Suppose you are a movie lover and you want to get the list of top 5 movies from 10 websites. One way will be to visit all 10 websites and see the top 5 list. This method though is used in general by lot of people but its quite tiring method. It will take you 10-15 minutes to browse all the websites and see the top 5 list of movies. One easy way will be if those movie websites provides RSS feeds to be used by the users. If they provide RSS feeds you can embed them in your page and now you don't have to browse all the websites since the information is available on a single page. Hence, this saves you a time and a lot of browsing.
Most of the Blogs websites provide RSS feeds so you can embed your or someone's else latest entries of blog on your website. In this article we will see how we can embed RSS feeds to our webform using Asp.net.
Most of the Blogs websites provide RSS feeds so you can embed your or someone's else latest entries of blog on your website. In this article we will see how we can embed RSS feeds to our webform using Asp.net.
Friday, April 18, 2008
Serial Communication with MSCommLib for GSM
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Text;
using System.IO;
using System.Diagnostics;
using System.Threading;
namespace Serial_Demo
{
///
/// Summary description for Form1.
///
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.RichTextBox rtfTerminal;
private System.Windows.Forms.Button btn_dial;
private AxMSCommLib.AxMSComm com;
private System.Windows.Forms.Label label6;
private System.Windows.Forms.TextBox txt_phoneno;
private System.Windows.Forms.Button btn_disconnect;
private System.Windows.Forms.Label label7;
private System.Windows.Forms.TextBox txt_sendmessage;
private System.Windows.Forms.Button btn_sendmessage;
private System.Windows.Forms.Label label8;
private System.Windows.Forms.Label Message;
private System.Windows.Forms.Panel panel1;
public Form1()
{
InitializeComponent();
// Initialize the COM Port control
InitComPort();
}
private void InitComPort()
{
// Set the com port to be 1
com.CommPort = 1;
// This port is already open, then close.
if (com.PortOpen)
com.PortOpen=false;
// Trigger the OnComm event whenever data is received
com.RThreshold = 1;
// Set the port to 9600 baud, no parity bit, 8 data bits, 1 stop bit (all standard)
com.Settings = "9600,n,8,1";
// Force the DTR line high, used sometimes to hang up modems
//com.DTREnable = true;
com.RTSEnable=true;
// No handshaking is used
com.Handshaking = MSCommLib.HandshakeConstants.comNone;
// Use this line instead for byte array input, best for most communications
com.InputMode = MSCommLib.InputModeConstants.comInputModeText;
// Read the entire waiting data when com.Input is used
com.InputLen = 0;
// Don't discard nulls, 0x00 is a useful byte
com.NullDiscard = false;
// Attach the event handler
com.OnComm += new System.EventHandler(this.OnComm);
com.PortOpen = true;
}
private void OnComm(object sender, EventArgs e) // MSCommLib OnComm Event Handler
{
// Wait for Some mili-seconds then process
// The response.
Thread.Sleep(200);
if (com.InBufferCount > 0)
{
try
{
// If you want to receive data in Binary mode
// Remove below 2 comment lines
// And comment lines for Process response in
// Text mode.
//byte[] b1=(byte[])com.Input;
//ProcessResponseBinary(b1);
// Process response in Text mode.
string response=(string)com.Input;
ProcessResponseText(response);
}
catch(Exception ex)
{
MessageBox.Show(ex.Message, this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}
}
// If you receive binary data as response.
private void ProcessResponseBinary(byte[] response)
{
for(int i=0; i< response.Length; i++)
{
rtfTerminal.AppendText(response[i].ToString() + " ");
}
rtfTerminal.AppendText("\n");
}
// If you receive Text data as response
private void ProcessResponseText(string input)
{
// Send incoming data to a Rich Text Box
if( input.Trim().Equals("RING"))
{
Message.Text="Ring...";
}
else
if( input.Trim().Equals("CONNECT 9600"))
{
MessageBox.Show(input.Trim(), this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
}
else
{
MessageBox.Show(input.Trim(), this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
Message.Text=input.Trim();
}
// Append output response to RichText Box
rtfTerminal.AppendText(input + "\n");
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
///
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
///
private void InitializeComponent()
{
System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(Form1));
this.rtfTerminal = new System.Windows.Forms.RichTextBox();
this.btn_dial = new System.Windows.Forms.Button();
this.com = new AxMSCommLib.AxMSComm();
this.label6 = new System.Windows.Forms.Label();
this.txt_phoneno = new System.Windows.Forms.TextBox();
this.btn_disconnect = new System.Windows.Forms.Button();
this.Message = new System.Windows.Forms.Label();
this.label7 = new System.Windows.Forms.Label();
this.txt_sendmessage = new System.Windows.Forms.TextBox();
this.btn_sendmessage = new System.Windows.Forms.Button();
this.label8 = new System.Windows.Forms.Label();
this.panel1 = new System.Windows.Forms.Panel();
((System.ComponentModel.ISupportInitialize)(this.com)).BeginInit();
this.panel1.SuspendLayout();
this.SuspendLayout();
//
// rtfTerminal
//
this.rtfTerminal.Location = new System.Drawing.Point(0, 136);
this.rtfTerminal.Name = "rtfTerminal";
this.rtfTerminal.Size = new System.Drawing.Size(280, 112);
this.rtfTerminal.TabIndex = 1;
this.rtfTerminal.Text = "";
//
// btn_dial
//
this.btn_dial.Location = new System.Drawing.Point(8, 72);
this.btn_dial.Name = "btn_dial";
this.btn_dial.TabIndex = 8;
this.btn_dial.Text = "Dial";
this.btn_dial.Click += new System.EventHandler(this.btn_dial_Click);
//
// com
//
this.com.Enabled = true;
this.com.Location = new System.Drawing.Point(160, 104);
this.com.Name = "com";
this.com.Size = new System.Drawing.Size(38, 38);
this.com.TabIndex = 14;
//
// label6
//
this.label6.BackColor = System.Drawing.Color.Transparent;
this.label6.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.label6.ForeColor = System.Drawing.SystemColors.Highlight;
this.label6.Location = new System.Drawing.Point(8, 8);
this.label6.Name = "label6";
this.label6.Size = new System.Drawing.Size(64, 23);
this.label6.TabIndex = 15;
this.label6.Text = "GSM No:";
//
// txt_phoneno
//
this.txt_phoneno.Location = new System.Drawing.Point(88, 8);
this.txt_phoneno.Name = "txt_phoneno";
this.txt_phoneno.Size = new System.Drawing.Size(168, 20);
this.txt_phoneno.TabIndex = 16;
this.txt_phoneno.Text = "";
//
// btn_disconnect
//
this.btn_disconnect.Location = new System.Drawing.Point(184, 72);
this.btn_disconnect.Name = "btn_disconnect";
this.btn_disconnect.TabIndex = 17;
this.btn_disconnect.Text = "Disconnect";
this.btn_disconnect.Click += new System.EventHandler(this.btn_disconnect_Click);
//
// Message
//
this.Message.BackColor = System.Drawing.Color.Transparent;
this.Message.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.Message.ForeColor = System.Drawing.Color.MidnightBlue;
this.Message.Location = new System.Drawing.Point(0, 248);
this.Message.Name = "Message";
this.Message.Size = new System.Drawing.Size(280, 23);
this.Message.TabIndex = 18;
//
// label7
//
this.label7.BackColor = System.Drawing.Color.Transparent;
this.label7.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.label7.ForeColor = System.Drawing.SystemColors.Highlight;
this.label7.Location = new System.Drawing.Point(8, 40);
this.label7.Name = "label7";
this.label7.Size = new System.Drawing.Size(72, 32);
this.label7.TabIndex = 19;
this.label7.Text = "Message:";
//
// txt_sendmessage
//
this.txt_sendmessage.Location = new System.Drawing.Point(88, 40);
this.txt_sendmessage.Name = "txt_sendmessage";
this.txt_sendmessage.Size = new System.Drawing.Size(168, 20);
this.txt_sendmessage.TabIndex = 20;
this.txt_sendmessage.Text = "";
//
// btn_sendmessage
//
this.btn_sendmessage.Location = new System.Drawing.Point(96, 72);
this.btn_sendmessage.Name = "btn_sendmessage";
this.btn_sendmessage.TabIndex = 21;
this.btn_sendmessage.Text = "Send";
this.btn_sendmessage.Click += new System.EventHandler(this.btn_sendmessage_Click);
//
// label8
//
this.label8.BackColor = System.Drawing.Color.Transparent;
this.label8.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.label8.ForeColor = System.Drawing.SystemColors.Highlight;
this.label8.Location = new System.Drawing.Point(0, 112);
this.label8.Name = "label8";
this.label8.Size = new System.Drawing.Size(160, 24);
this.label8.TabIndex = 22;
this.label8.Text = "Received messages:";
//
// panel1
//
this.panel1.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.panel1.Controls.Add(this.btn_dial);
this.panel1.Controls.Add(this.btn_disconnect);
this.panel1.Controls.Add(this.txt_phoneno);
this.panel1.Controls.Add(this.label6);
this.panel1.Controls.Add(this.label7);
this.panel1.Controls.Add(this.txt_sendmessage);
this.panel1.Controls.Add(this.btn_sendmessage);
this.panel1.Location = new System.Drawing.Point(0, 0);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(280, 104);
this.panel1.TabIndex = 23;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(280, 269);
this.Controls.Add(this.panel1);
this.Controls.Add(this.label8);
this.Controls.Add(this.rtfTerminal);
this.Controls.Add(this.com);
this.Controls.Add(this.Message);
this.Name = "Form1";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "Serial Demo";
((System.ComponentModel.ISupportInitialize)(this.com)).EndInit();
this.panel1.ResumeLayout(false);
this.ResumeLayout(false);
}
#endregion
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void btn_dial_Click(object sender, System.EventArgs e)
{
if( txt_phoneno.Text.Trim().Equals(""))
{
MessageBox.Show("Please Specify Phone Number", this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
txt_phoneno.Focus();
return;
}
if(! com.PortOpen )
com.PortOpen=true;
// GSM Command Dial a Modem
// ATD\n
string gsm_command="ATD";
string phone_number=txt_phoneno.Text.Trim();
string command1=gsm_command + phone_number + "\n";
byte[] command_to_dial=System.Text.ASCIIEncoding.Default.GetBytes(command1);
com.Output=command_to_dial;
Message.Text="Dialing...";
}
private void btn_disconnect_Click(object sender, System.EventArgs e)
{
// If com port is open then close it
// to disconnect connection
if( com.PortOpen )
{
com.PortOpen=false;
MessageBox.Show("Disconnected...", this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
Message.Text="";
rtfTerminal.Text="";
}
}
private void btn_sendmessage_Click(object sender, System.EventArgs e)
{
string msg="";
if( txt_sendmessage.Text.Trim().Equals(""))
{
MessageBox.Show("Please Specify Command", this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
txt_sendmessage.Focus();
return;
}
if(! com.PortOpen )
com.PortOpen=true;
// To send text messages
// If you are using GSM Modem and you want to send
// Command then use GetByes of your message
// To send Byte data from com port
msg=txt_sendmessage.Text.Trim() + "\n";
com.Output = System.Text.ASCIIEncoding.Default.GetBytes(msg);
// Or Else If systems are connected with Serial
// Cable, Output simple text directly
// com.Output= txt_sendmessage.Text;
Message.Text="Message Sent....";
}
}
}
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Text;
using System.IO;
using System.Diagnostics;
using System.Threading;
namespace Serial_Demo
{
///
/// Summary description for Form1.
///
public class Form1 : System.Windows.Forms.Form
{
private System.Windows.Forms.RichTextBox rtfTerminal;
private System.Windows.Forms.Button btn_dial;
private AxMSCommLib.AxMSComm com;
private System.Windows.Forms.Label label6;
private System.Windows.Forms.TextBox txt_phoneno;
private System.Windows.Forms.Button btn_disconnect;
private System.Windows.Forms.Label label7;
private System.Windows.Forms.TextBox txt_sendmessage;
private System.Windows.Forms.Button btn_sendmessage;
private System.Windows.Forms.Label label8;
private System.Windows.Forms.Label Message;
private System.Windows.Forms.Panel panel1;
public Form1()
{
InitializeComponent();
// Initialize the COM Port control
InitComPort();
}
private void InitComPort()
{
// Set the com port to be 1
com.CommPort = 1;
// This port is already open, then close.
if (com.PortOpen)
com.PortOpen=false;
// Trigger the OnComm event whenever data is received
com.RThreshold = 1;
// Set the port to 9600 baud, no parity bit, 8 data bits, 1 stop bit (all standard)
com.Settings = "9600,n,8,1";
// Force the DTR line high, used sometimes to hang up modems
//com.DTREnable = true;
com.RTSEnable=true;
// No handshaking is used
com.Handshaking = MSCommLib.HandshakeConstants.comNone;
// Use this line instead for byte array input, best for most communications
com.InputMode = MSCommLib.InputModeConstants.comInputModeText;
// Read the entire waiting data when com.Input is used
com.InputLen = 0;
// Don't discard nulls, 0x00 is a useful byte
com.NullDiscard = false;
// Attach the event handler
com.OnComm += new System.EventHandler(this.OnComm);
com.PortOpen = true;
}
private void OnComm(object sender, EventArgs e) // MSCommLib OnComm Event Handler
{
// Wait for Some mili-seconds then process
// The response.
Thread.Sleep(200);
if (com.InBufferCount > 0)
{
try
{
// If you want to receive data in Binary mode
// Remove below 2 comment lines
// And comment lines for Process response in
// Text mode.
//byte[] b1=(byte[])com.Input;
//ProcessResponseBinary(b1);
// Process response in Text mode.
string response=(string)com.Input;
ProcessResponseText(response);
}
catch(Exception ex)
{
MessageBox.Show(ex.Message, this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
}
}
}
// If you receive binary data as response.
private void ProcessResponseBinary(byte[] response)
{
for(int i=0; i< response.Length; i++)
{
rtfTerminal.AppendText(response[i].ToString() + " ");
}
rtfTerminal.AppendText("\n");
}
// If you receive Text data as response
private void ProcessResponseText(string input)
{
// Send incoming data to a Rich Text Box
if( input.Trim().Equals("RING"))
{
Message.Text="Ring...";
}
else
if( input.Trim().Equals("CONNECT 9600"))
{
MessageBox.Show(input.Trim(), this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
}
else
{
MessageBox.Show(input.Trim(), this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
Message.Text=input.Trim();
}
// Append output response to RichText Box
rtfTerminal.AppendText(input + "\n");
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
///
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
///
private void InitializeComponent()
{
System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(Form1));
this.rtfTerminal = new System.Windows.Forms.RichTextBox();
this.btn_dial = new System.Windows.Forms.Button();
this.com = new AxMSCommLib.AxMSComm();
this.label6 = new System.Windows.Forms.Label();
this.txt_phoneno = new System.Windows.Forms.TextBox();
this.btn_disconnect = new System.Windows.Forms.Button();
this.Message = new System.Windows.Forms.Label();
this.label7 = new System.Windows.Forms.Label();
this.txt_sendmessage = new System.Windows.Forms.TextBox();
this.btn_sendmessage = new System.Windows.Forms.Button();
this.label8 = new System.Windows.Forms.Label();
this.panel1 = new System.Windows.Forms.Panel();
((System.ComponentModel.ISupportInitialize)(this.com)).BeginInit();
this.panel1.SuspendLayout();
this.SuspendLayout();
//
// rtfTerminal
//
this.rtfTerminal.Location = new System.Drawing.Point(0, 136);
this.rtfTerminal.Name = "rtfTerminal";
this.rtfTerminal.Size = new System.Drawing.Size(280, 112);
this.rtfTerminal.TabIndex = 1;
this.rtfTerminal.Text = "";
//
// btn_dial
//
this.btn_dial.Location = new System.Drawing.Point(8, 72);
this.btn_dial.Name = "btn_dial";
this.btn_dial.TabIndex = 8;
this.btn_dial.Text = "Dial";
this.btn_dial.Click += new System.EventHandler(this.btn_dial_Click);
//
// com
//
this.com.Enabled = true;
this.com.Location = new System.Drawing.Point(160, 104);
this.com.Name = "com";
this.com.Size = new System.Drawing.Size(38, 38);
this.com.TabIndex = 14;
//
// label6
//
this.label6.BackColor = System.Drawing.Color.Transparent;
this.label6.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.label6.ForeColor = System.Drawing.SystemColors.Highlight;
this.label6.Location = new System.Drawing.Point(8, 8);
this.label6.Name = "label6";
this.label6.Size = new System.Drawing.Size(64, 23);
this.label6.TabIndex = 15;
this.label6.Text = "GSM No:";
//
// txt_phoneno
//
this.txt_phoneno.Location = new System.Drawing.Point(88, 8);
this.txt_phoneno.Name = "txt_phoneno";
this.txt_phoneno.Size = new System.Drawing.Size(168, 20);
this.txt_phoneno.TabIndex = 16;
this.txt_phoneno.Text = "";
//
// btn_disconnect
//
this.btn_disconnect.Location = new System.Drawing.Point(184, 72);
this.btn_disconnect.Name = "btn_disconnect";
this.btn_disconnect.TabIndex = 17;
this.btn_disconnect.Text = "Disconnect";
this.btn_disconnect.Click += new System.EventHandler(this.btn_disconnect_Click);
//
// Message
//
this.Message.BackColor = System.Drawing.Color.Transparent;
this.Message.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.Message.ForeColor = System.Drawing.Color.MidnightBlue;
this.Message.Location = new System.Drawing.Point(0, 248);
this.Message.Name = "Message";
this.Message.Size = new System.Drawing.Size(280, 23);
this.Message.TabIndex = 18;
//
// label7
//
this.label7.BackColor = System.Drawing.Color.Transparent;
this.label7.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.label7.ForeColor = System.Drawing.SystemColors.Highlight;
this.label7.Location = new System.Drawing.Point(8, 40);
this.label7.Name = "label7";
this.label7.Size = new System.Drawing.Size(72, 32);
this.label7.TabIndex = 19;
this.label7.Text = "Message:";
//
// txt_sendmessage
//
this.txt_sendmessage.Location = new System.Drawing.Point(88, 40);
this.txt_sendmessage.Name = "txt_sendmessage";
this.txt_sendmessage.Size = new System.Drawing.Size(168, 20);
this.txt_sendmessage.TabIndex = 20;
this.txt_sendmessage.Text = "";
//
// btn_sendmessage
//
this.btn_sendmessage.Location = new System.Drawing.Point(96, 72);
this.btn_sendmessage.Name = "btn_sendmessage";
this.btn_sendmessage.TabIndex = 21;
this.btn_sendmessage.Text = "Send";
this.btn_sendmessage.Click += new System.EventHandler(this.btn_sendmessage_Click);
//
// label8
//
this.label8.BackColor = System.Drawing.Color.Transparent;
this.label8.Font = new System.Drawing.Font("Microsoft Sans Serif", 8.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.label8.ForeColor = System.Drawing.SystemColors.Highlight;
this.label8.Location = new System.Drawing.Point(0, 112);
this.label8.Name = "label8";
this.label8.Size = new System.Drawing.Size(160, 24);
this.label8.TabIndex = 22;
this.label8.Text = "Received messages:";
//
// panel1
//
this.panel1.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.panel1.Controls.Add(this.btn_dial);
this.panel1.Controls.Add(this.btn_disconnect);
this.panel1.Controls.Add(this.txt_phoneno);
this.panel1.Controls.Add(this.label6);
this.panel1.Controls.Add(this.label7);
this.panel1.Controls.Add(this.txt_sendmessage);
this.panel1.Controls.Add(this.btn_sendmessage);
this.panel1.Location = new System.Drawing.Point(0, 0);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(280, 104);
this.panel1.TabIndex = 23;
//
// Form1
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(280, 269);
this.Controls.Add(this.panel1);
this.Controls.Add(this.label8);
this.Controls.Add(this.rtfTerminal);
this.Controls.Add(this.com);
this.Controls.Add(this.Message);
this.Name = "Form1";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "Serial Demo";
((System.ComponentModel.ISupportInitialize)(this.com)).EndInit();
this.panel1.ResumeLayout(false);
this.ResumeLayout(false);
}
#endregion
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run(new Form1());
}
private void btn_dial_Click(object sender, System.EventArgs e)
{
if( txt_phoneno.Text.Trim().Equals(""))
{
MessageBox.Show("Please Specify Phone Number", this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
txt_phoneno.Focus();
return;
}
if(! com.PortOpen )
com.PortOpen=true;
// GSM Command Dial a Modem
// ATD
string gsm_command="ATD";
string phone_number=txt_phoneno.Text.Trim();
string command1=gsm_command + phone_number + "\n";
byte[] command_to_dial=System.Text.ASCIIEncoding.Default.GetBytes(command1);
com.Output=command_to_dial;
Message.Text="Dialing...";
}
private void btn_disconnect_Click(object sender, System.EventArgs e)
{
// If com port is open then close it
// to disconnect connection
if( com.PortOpen )
{
com.PortOpen=false;
MessageBox.Show("Disconnected...", this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
Message.Text="";
rtfTerminal.Text="";
}
}
private void btn_sendmessage_Click(object sender, System.EventArgs e)
{
string msg="";
if( txt_sendmessage.Text.Trim().Equals(""))
{
MessageBox.Show("Please Specify Command", this.Text,
MessageBoxButtons.OK,MessageBoxIcon.Information);
txt_sendmessage.Focus();
return;
}
if(! com.PortOpen )
com.PortOpen=true;
// To send text messages
// If you are using GSM Modem and you want to send
// Command then use GetByes of your message
// To send Byte data from com port
msg=txt_sendmessage.Text.Trim() + "\n";
com.Output = System.Text.ASCIIEncoding.Default.GetBytes(msg);
// Or Else If systems are connected with Serial
// Cable, Output simple text directly
// com.Output= txt_sendmessage.Text;
Message.Text="Message Sent....";
}
}
}
A solution for inserting ,updating ,deleting database with class and stored procedure
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public class Class1
{
SqlConnection scn = new SqlConnection("Data Source=.;Initial Catalog=Routingmail;Integrated Security=True");
public Class1()
{
}
public void prc(string prcname, string[] prcnames, string[] prcvalues)
{
scn.Open();
SqlCommand cmd = new SqlCommand(prcname, scn);
cmd.CommandType = CommandType.StoredProcedure;
for(int i = 0; i <= prcnames.Length - 1; i++)
{
cmd.Parameters.Add(prcnames[i], prcvalues[i]);
}
cmd.ExecuteNonQuery();
scn.Close();
}
}
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public class Class1
{
SqlConnection scn = new SqlConnection("Data Source=.;Initial Catalog=Routingmail;Integrated Security=True");
public Class1()
{
}
public void prc(string prcname, string[] prcnames, string[] prcvalues)
{
scn.Open();
SqlCommand cmd = new SqlCommand(prcname, scn);
cmd.CommandType = CommandType.StoredProcedure;
for(int i = 0; i <= prcnames.Length - 1; i++)
{
cmd.Parameters.Add(prcnames[i], prcvalues[i]);
}
cmd.ExecuteNonQuery();
scn.Close();
}
}
Simple Code for Change Password
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public partial class ChangePassword : System.Web.UI.Page
{
SqlConnection scn = new SqlConnection("Data Source=.;Initial Catalog=Routingmail;Integrated Security=True");
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
scn.Open();
SqlCommand cmd = new SqlCommand("select pwd from Registration where userid='" + Session["userid"].ToString() + "'", scn);
string str = Convert.ToString(cmd.ExecuteScalar());
txtname.Text = str;
scn.Close();
}
}
protected void btnsubmit_Click(object sender, EventArgs e)
{
if (scn.State == ConnectionState.Closed)
{
scn.Open();
}
SqlCommand cmd = new SqlCommand("update Registration set pwd='" + txtpassword.Text + "' where userid='" + Session["userid"].ToString() + "'", scn);
cmd.ExecuteNonQuery();
scn.Close();
}
}
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
public partial class ChangePassword : System.Web.UI.Page
{
SqlConnection scn = new SqlConnection("Data Source=.;Initial Catalog=Routingmail;Integrated Security=True");
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
scn.Open();
SqlCommand cmd = new SqlCommand("select pwd from Registration where userid='" + Session["userid"].ToString() + "'", scn);
string str = Convert.ToString(cmd.ExecuteScalar());
txtname.Text = str;
scn.Close();
}
}
protected void btnsubmit_Click(object sender, EventArgs e)
{
if (scn.State == ConnectionState.Closed)
{
scn.Open();
}
SqlCommand cmd = new SqlCommand("update Registration set pwd='" + txtpassword.Text + "' where userid='" + Session["userid"].ToString() + "'", scn);
cmd.ExecuteNonQuery();
scn.Close();
}
}
Simple Code for Forgot Password
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data .SqlClient;
public partial class Forgotpwd : System.Web.UI.Page
{
SqlConnection scn = new SqlConnection("Data source=.;Initial Catalog=Routingmail;Integrated Security=True");
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
TextBox1.Text = Session["userid"].ToString();
if (scn.State == ConnectionState.Closed)
{
scn.Open();
}
SqlCommand cmd = new SqlCommand("Select secquestion from registration where userid='"+TextBox1.Text+"'",scn);
string str = Convert.ToString(cmd.ExecuteScalar());
txtsecuriy.Text = str;
}
}
protected void Button1_Click(object sender, EventArgs e)
{
scn.Open();
SqlCommand cmd = new SqlCommand("select pwd from registration where answer='" + txtanswer.Text + "'", scn);
String str = Convert.ToString(cmd.ExecuteScalar());
Label1.Text = "your password is " + str;
Label1.Visible = true;
scn.Close();
}
}
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data .SqlClient;
public partial class Forgotpwd : System.Web.UI.Page
{
SqlConnection scn = new SqlConnection("Data source=.;Initial Catalog=Routingmail;Integrated Security=True");
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
TextBox1.Text = Session["userid"].ToString();
if (scn.State == ConnectionState.Closed)
{
scn.Open();
}
SqlCommand cmd = new SqlCommand("Select secquestion from registration where userid='"+TextBox1.Text+"'",scn);
string str = Convert.ToString(cmd.ExecuteScalar());
txtsecuriy.Text = str;
}
}
protected void Button1_Click(object sender, EventArgs e)
{
scn.Open();
SqlCommand cmd = new SqlCommand("select pwd from registration where answer='" + txtanswer.Text + "'", scn);
String str = Convert.ToString(cmd.ExecuteScalar());
Label1.Text = "your password is " + str;
Label1.Visible = true;
scn.Close();
}
}
Simple Code for login
protected void btnsubmit_Click(object sender, EventArgs e)
{
scn.Open();
SqlCommand cmd = new SqlCommand("select pwd from Registration where userid='" + txtname.Text + "'", scn);
string str = Convert.ToString(cmd.ExecuteScalar());
if (str == "")
{
Label1.Visible = true;
Label1.Text = "Login failed";
txtname.Text = "";
txtpassword.Text = "";
}
else
{
if (txtpassword.Text == str)
{
Session["userid"] = txtname.Text;
Session["password"] = txtpassword.Text;
Response.Redirect("mailrouting.aspx");
Label1.Visible = false;
txtname.Text = "";
txtpassword.Text = "";
Response.Redirect("mailrouting.aspx");
}
else
{
Label1.Visible = true;
Label1.Text = "Incorrect Password";
txtname.Text = "";
txtpassword.Text = "";
}
}
scn.Close();
}
{
scn.Open();
SqlCommand cmd = new SqlCommand("select pwd from Registration where userid='" + txtname.Text + "'", scn);
string str = Convert.ToString(cmd.ExecuteScalar());
if (str == "")
{
Label1.Visible = true;
Label1.Text = "Login failed";
txtname.Text = "";
txtpassword.Text = "";
}
else
{
if (txtpassword.Text == str)
{
Session["userid"] = txtname.Text;
Session["password"] = txtpassword.Text;
Response.Redirect("mailrouting.aspx");
Label1.Visible = false;
txtname.Text = "";
txtpassword.Text = "";
Response.Redirect("mailrouting.aspx");
}
else
{
Label1.Visible = true;
Label1.Text = "Incorrect Password";
txtname.Text = "";
txtpassword.Text = "";
}
}
scn.Close();
}
A smple Code for Mail Routing
using System;
using System.Data;
using aspNetEmail;
using aspNetPOP3;
using aspNetMime;
using AdvancedIntellect;
using System.Data.SqlClient;
using System.Data.SqlTypes;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Text;
using System.Net.Sockets;
public partial class mailrouting : System.Web.UI.Page
{
POP3 pop;
// POP3ConnectionException ex = new POP3ConnectionException();
string primary, primarypass, mail1, mailpass1, mail2, mailpass2, id, pwd;
int mcount = 0;
SqlConnection con = new SqlConnection("Data Source=.;Initial Catalog=Routingmail;Integrated Security=True");
protected void Page_Load(object sender, EventArgs e)
{
con.Open();
String userid = Session["userid"].ToString();
SqlCommand cmd = new SqlCommand("select * from phoneemail where userid='" + userid + "'", con);
SqlDataReader rd = cmd.ExecuteReader();
if (rd.Read())
{
primary = rd[1].ToString();
primarypass = rd[2].ToString();
mail1 = rd[4].ToString();
mailpass1 = rd[5].ToString();
mail2 = rd[6].ToString();
mailpass2 = rd[7].ToString();
}
rd.Close();
con.Close();
for (int i = 1; i < 4; i++)
{
switch (i)
{
case 1:
id = mail1;
pwd = mailpass1;
break;
case 2:
id = mail2;
pwd = mailpass2;
break;
default:
break;
}
string ab = id.Substring(id.IndexOf('@') + 1);
if (ab.Equals("gmail.com"))
{
pop = new POP3("pop.gmail.com", id, pwd);
AdvancedIntellect.Ssl.SslSocket ss1 = new AdvancedIntellect.Ssl.SslSocket();
pop.LoadSslSocket(ss1);
pop.Port = 995;
}
else
{
pop = new POP3("mail.ideonics.co.in", id, pwd);
}
pop.LogInMemory = true;
pop.Connect();
//pop.Connect(id, pwd, "mail.ideonics.co.in");
pop.PopulateInboxStats();
int count = pop.MessageCount();
if (count != 0)
{
for (int j = 0; j < count; j++)
{
try
{
if (pop.GetMessage(j).Subject != null)
{
if (!pop.GetMessage(j).Subject.Value.Equals("Reply"))
{
MimeMessage m = pop.GetMessage(j);
forward(m);
Reply(m.Subject.Value);
pop.Delete(j);
Label2.Text = "Mails are sending............" + mcount;
}
}
}
catch (Exception ex)
{
Response.Write(ex.Message);
break;
}
}
Label2.Text = "Total Mails sent................." + mcount;
pop.Disconnect();
con.Close();
}
}
}
public void forward(MimeMessage m)
{
mcount++;
StringBuilder inlineEmail = new StringBuilder();
inlineEmail.Append("---------- original Message---------\r\n");
if (m.From != null)
{
inlineEmail.Append("From:" + m.From.ToString() + "\r\n");
}
if ((m.To != null) && (m.To.Count > 0))
{
inlineEmail.Append("to:" + m.To.ToString() + "\r\n");
}
if (m.Date != null)
{
inlineEmail.Append("Date:" + m.Date + "\r\n");
}
if (m.Subject != null)
{
inlineEmail.Append("SUBJECT: " + aspNetMime.Header.DeocodeHeaderValue(m.Subject.Value) + "\r\n");
}
if (m.TextMimePart != null)
{
inlineEmail.Append("\r\n");
inlineEmail.Append(m.TextMimePart.DecodedText());
}
MimePartCollection attachments = m.Attachments;
EmailMessage email = new EmailMessage();
email.Server = "mail.ideonics.co.in";
email.FromAddress = id;
email.To = primary;
email.Subject = "Mail from" + id;
email.Body = "see email below\r\n\r\n";
email.Body += inlineEmail.ToString();
if ((attachments != null) && (attachments.Count > 0))
{
for (int i = 0; i < attachments.Count; i++)
{
//add each attachment to the email message
Attachment a = new Attachment(attachments[i].Data(), attachments[i].AttachmentName());
email.AddAttachment(a);
}
}
email.Send();
}
public void Reply(string sub)
{
EmailMessage email = new EmailMessage();
email.Server = "mail.ideonics.co.in";
email.FromAddress = primary;
email.To = id;
email.Subject = "Reply";
email.Body = sub + "is forwardedto your primary id" + primary;
email.Send();
}
}
using System.Data;
using aspNetEmail;
using aspNetPOP3;
using aspNetMime;
using AdvancedIntellect;
using System.Data.SqlClient;
using System.Data.SqlTypes;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Text;
using System.Net.Sockets;
public partial class mailrouting : System.Web.UI.Page
{
POP3 pop;
// POP3ConnectionException ex = new POP3ConnectionException();
string primary, primarypass, mail1, mailpass1, mail2, mailpass2, id, pwd;
int mcount = 0;
SqlConnection con = new SqlConnection("Data Source=.;Initial Catalog=Routingmail;Integrated Security=True");
protected void Page_Load(object sender, EventArgs e)
{
con.Open();
String userid = Session["userid"].ToString();
SqlCommand cmd = new SqlCommand("select * from phoneemail where userid='" + userid + "'", con);
SqlDataReader rd = cmd.ExecuteReader();
if (rd.Read())
{
primary = rd[1].ToString();
primarypass = rd[2].ToString();
mail1 = rd[4].ToString();
mailpass1 = rd[5].ToString();
mail2 = rd[6].ToString();
mailpass2 = rd[7].ToString();
}
rd.Close();
con.Close();
for (int i = 1; i < 4; i++)
{
switch (i)
{
case 1:
id = mail1;
pwd = mailpass1;
break;
case 2:
id = mail2;
pwd = mailpass2;
break;
default:
break;
}
string ab = id.Substring(id.IndexOf('@') + 1);
if (ab.Equals("gmail.com"))
{
pop = new POP3("pop.gmail.com", id, pwd);
AdvancedIntellect.Ssl.SslSocket ss1 = new AdvancedIntellect.Ssl.SslSocket();
pop.LoadSslSocket(ss1);
pop.Port = 995;
}
else
{
pop = new POP3("mail.ideonics.co.in", id, pwd);
}
pop.LogInMemory = true;
pop.Connect();
//pop.Connect(id, pwd, "mail.ideonics.co.in");
pop.PopulateInboxStats();
int count = pop.MessageCount();
if (count != 0)
{
for (int j = 0; j < count; j++)
{
try
{
if (pop.GetMessage(j).Subject != null)
{
if (!pop.GetMessage(j).Subject.Value.Equals("Reply"))
{
MimeMessage m = pop.GetMessage(j);
forward(m);
Reply(m.Subject.Value);
pop.Delete(j);
Label2.Text = "Mails are sending............" + mcount;
}
}
}
catch (Exception ex)
{
Response.Write(ex.Message);
break;
}
}
Label2.Text = "Total Mails sent................." + mcount;
pop.Disconnect();
con.Close();
}
}
}
public void forward(MimeMessage m)
{
mcount++;
StringBuilder inlineEmail = new StringBuilder();
inlineEmail.Append("---------- original Message---------\r\n");
if (m.From != null)
{
inlineEmail.Append("From:" + m.From.ToString() + "\r\n");
}
if ((m.To != null) && (m.To.Count > 0))
{
inlineEmail.Append("to:" + m.To.ToString() + "\r\n");
}
if (m.Date != null)
{
inlineEmail.Append("Date:" + m.Date + "\r\n");
}
if (m.Subject != null)
{
inlineEmail.Append("SUBJECT: " + aspNetMime.Header.DeocodeHeaderValue(m.Subject.Value) + "\r\n");
}
if (m.TextMimePart != null)
{
inlineEmail.Append("\r\n");
inlineEmail.Append(m.TextMimePart.DecodedText());
}
MimePartCollection attachments = m.Attachments;
EmailMessage email = new EmailMessage();
email.Server = "mail.ideonics.co.in";
email.FromAddress = id;
email.To = primary;
email.Subject = "Mail from" + id;
email.Body = "see email below\r\n\r\n";
email.Body += inlineEmail.ToString();
if ((attachments != null) && (attachments.Count > 0))
{
for (int i = 0; i < attachments.Count; i++)
{
//add each attachment to the email message
Attachment a = new Attachment(attachments[i].Data(), attachments[i].AttachmentName());
email.AddAttachment(a);
}
}
email.Send();
}
public void Reply(string sub)
{
EmailMessage email = new EmailMessage();
email.Server = "mail.ideonics.co.in";
email.FromAddress = primary;
email.To = id;
email.Subject = "Reply";
email.Body = sub + "is forwardedto your primary id" + primary;
email.Send();
}
}
A simple code for Check Availability
protected void btncheckavail_Click(object sender, EventArgs e)
{
scn.Open();
SqlCommand cmd = new SqlCommand("select userid from Registration where userid='" + txtuserid.Text + "'", scn);
string str = Convert.ToString(cmd.ExecuteScalar());
if (str =="")
{
lbl.Text = UserID is Available
}
else
{
lbl.Text = "This UserID is Already Exists";
lbl.Visible = true;
}
}
{
scn.Open();
SqlCommand cmd = new SqlCommand("select userid from Registration where userid='" + txtuserid.Text + "'", scn);
string str = Convert.ToString(cmd.ExecuteScalar());
if (str =="")
{
lbl.Text = UserID is Available
}
else
{
lbl.Text = "This UserID is Already Exists";
lbl.Visible = true;
}
}
Serial Communication with GSM in asp.net with c#
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.IO.Ports;
using System.IO;
using System.Threading;
using System.Transactions;
using System.Runtime.InteropServices.ComTypes;
using System.Text;
public partial class _Default : System.Web.UI.Page
{
SerialPort port = new SerialPort("COM2", 9600, Parity.None, 8, StopBits.One);
protected void Page_Load(object sender, EventArgs e)
{
port.RtsEnable = true;
port.DtrEnable = true;
port.Open();
port.Write("AT+CMGF=1" + "\r\n");
Thread.Sleep(2000);
string I = port.ReadExisting().ToString();
Thread.Sleep(2000);
port.Write("AT+CNMI=1,3,2,1" + "\r\n");
Thread.Sleep(2000);
string L = port.ReadExisting().ToString();
Thread.Sleep(2000);
port.Write("AT+CMGR=1" + "\r\n");
Thread.Sleep(2000);
string msg = port.ReadExisting().ToString();
Thread.Sleep(2000);
port.Write("AT+CMGS="+"+919961365059"+""+"\r\n" + "hai how are u" + "\r\n");
Thread.Sleep(2000);
string res = port.ReadExisting().ToString();
Thread.Sleep(2000);
int num = Convert.ToInt32("26");
string st = num.ToString("X");
port.Write(st+ "\r\n");
Thread.Sleep(2000);
string message = port.ReadExisting().ToString();
string u = b.Substring(0, message.IndexOf("\r\n"));
port.Close();
}
}
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.IO.Ports;
using System.IO;
using System.Threading;
using System.Transactions;
using System.Runtime.InteropServices.ComTypes;
using System.Text;
public partial class _Default : System.Web.UI.Page
{
SerialPort port = new SerialPort("COM2", 9600, Parity.None, 8, StopBits.One);
protected void Page_Load(object sender, EventArgs e)
{
port.RtsEnable = true;
port.DtrEnable = true;
port.Open();
port.Write("AT+CMGF=1" + "\r\n");
Thread.Sleep(2000);
string I = port.ReadExisting().ToString();
Thread.Sleep(2000);
port.Write("AT+CNMI=1,3,2,1" + "\r\n");
Thread.Sleep(2000);
string L = port.ReadExisting().ToString();
Thread.Sleep(2000);
port.Write("AT+CMGR=1" + "\r\n");
Thread.Sleep(2000);
string msg = port.ReadExisting().ToString();
Thread.Sleep(2000);
port.Write("AT+CMGS="+"+919961365059"+""+"\r\n" + "hai how are u" + "\r\n");
Thread.Sleep(2000);
string res = port.ReadExisting().ToString();
Thread.Sleep(2000);
int num = Convert.ToInt32("26");
string st = num.ToString("X");
port.Write(st+ "\r\n");
Thread.Sleep(2000);
string message = port.ReadExisting().ToString();
string u = b.Substring(0, message.IndexOf("\r\n"));
port.Close();
}
}
C Sharp (programming language)
C# is an object-oriented programming language developed by Microsoft as part of the .NET initiative and later approved as a standard by ECMA (ECMA-334) and ISO (ISO/IEC 23270). Anders Hejlsberg leads development of the C# language, which has a procedural, object-oriented syntax based on C++ and includes influences from aspects of several other programming languages (most notably Delphi and Java) with a particular emphasis on simplification.
Subscribe to:
Posts (Atom)
Using Authorization with Swagger in ASP.NET Core
Create Solution like below LoginModel.cs using System.ComponentModel.DataAnnotations; namespace UsingAuthorizationWithSwagger.Models { ...
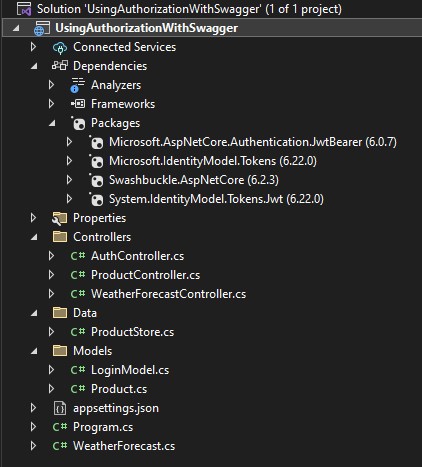
-
<ControlTemplate x:Key="TextBoxBaseControlTemplate" TargetType="{x:Type TextBoxBase}"> <Borde...
-
<?xml version="1.0" encoding="utf-8"?> <Report xmlns:rd="http://schemas.microsoft.com/SQLServer/reporting...
-
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.D...