using System.IO;
using System.IO.Compression;
FileStream sourceFile;
GZipStream compStream;
FileStream destFile;
protected void btnCompress_Click1(object sender, EventArgs e)
{
if (File1.PostedFile != null)
{
txtSource.Text = File1.PostedFile.FileName.ToString();
}
sourceFile = File.OpenRead(txtSource.Text);
destFile = File.Create(txtDestination.Text);
compStream = new GZipStream(destFile, CompressionMode.Compress);
int theByte = sourceFile.ReadByte();
while (theByte != -1)
{
compStream.WriteByte((byte)theByte);
theByte = sourceFile.ReadByte();
}
sourceFile.Close();
destFile.Close();
}
protected void btnDecompress_Click(object sender, EventArgs e)
{
if (File1.PostedFile != null)
{
txtSource.Text = File1.PostedFile.FileName.ToString();
}
string srcFile = txtSource.Text;
string dstFile = txtDestination.Text;
FileStream fsIn = null; // will open and read the srcFile
FileStream fsOut = null; // will be used by the GZipStream for output to the dstFile
GZipStream gzip = null;
const int bufferSize = 4096;
byte[] buffer = new byte[bufferSize];
int count = 0;
{
fsIn = new FileStream(srcFile, FileMode.Open, FileAccess.Read, FileShare.Read);
fsOut = new FileStream(dstFile, FileMode.Create, FileAccess.Write, FileShare.None);
gzip = new GZipStream(fsIn, CompressionMode.Decompress, true);
while (true)
{
count = gzip.Read(buffer, 0, bufferSize);
if (count != 0)
{
fsOut.Write(buffer, 0, count);
}
if (count != bufferSize)
{
// have reached the end
break;
}
}
}
}
}
ASP.NET is a development framework for building web pages and web sites with HTML, CSS, JavaScript and server scripting. ASP.NET supports three different development models: Web Pages, MVC (Model View Controller), and Web Forms.
Subscribe to:
Posts (Atom)
Using Authorization with Swagger in ASP.NET Core
Create Solution like below LoginModel.cs using System.ComponentModel.DataAnnotations; namespace UsingAuthorizationWithSwagger.Models { ...
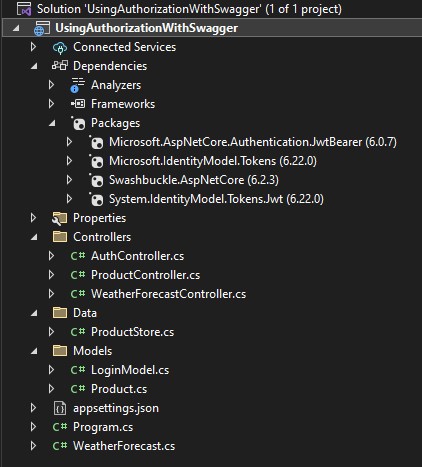
-
<ControlTemplate x:Key="TextBoxBaseControlTemplate" TargetType="{x:Type TextBoxBase}"> <Borde...
-
<?xml version="1.0" encoding="utf-8"?> <Report xmlns:rd="http://schemas.microsoft.com/SQLServer/reporting...
-
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.D...