private string dateConvert(string datDate){
System.Globalization.CultureInfo cultEnGb = new System.Globalization.CultureInfo("en-GB");
System.Globalization.CultureInfo cultEnUs = new System.Globalization.CultureInfo("en-US");
DateTime dtGb = Convert.ToDateTime(datDate, cultEnGb.DateTimeFormat); datDate = dtGb.ToString(cultEnUs.DateTimeFormat.ShortDatePattern);
return datDate;}
ASP.NET is a development framework for building web pages and web sites with HTML, CSS, JavaScript and server scripting. ASP.NET supports three different development models: Web Pages, MVC (Model View Controller), and Web Forms.
Tuesday, April 28, 2009
Monday, April 27, 2009
ASP.Net 2.0 C# DateTime IFormatProvider Using ParseExact
In the previous article C# Convert String to DateTime you learnt the use of Convert.ToDateTime function in C# ASP.Net 2.0. Following are 2 other methods to convert the DateTime format using IFormatProvider:
DateTime .ParseExact
DateTime.Parse
To use IFormatProvider you have to pass the culture info for DateTime format coz in different cultures DateTime format of displaying the sequence of month and date varies.
E.g.:
For French Culture use the following:
IFormatProvider culture = new CultureInfo("fr-Fr", true);
For US English use the following:
IFormatProvider culture = new CultureInfo("fr-Fr", true);
Both cultures mentioned above accept the different DateTime Formats.
If you are using
DateTime.Parse
then you have to pass the MM/dd/yyyy hh:mm:ss
Format of DateTime for French culture and dd/MM/yyyy hh:mm:ss for US English culture. You have to check the format for different cultures by changing the position of month and day.
Using DateTime Parse:
dt = DateTime.Parse(myDateTimeString, culture,DateTimeStyles.NoCurrentDateDefault);
Using DateTime ParseExact:
dt = DateTime.ParseExact(myDateTimeString,"MM/dd/yyyy hh:mm:ss", culture, DateTimeStyles.NoCurrentDateDefault);
C# Code to ParseExact the DateTime String:
string myDateTimeString;
myDateTimeString = "19/02/2008 05:44:00";
IFormatProvider culture = new CultureInfo("fr-Fr", true);
dt = DateTime.ParseExact(myDateTimeString,"dd/MM/yyyy hh:mm:ss", culture, DateTimeStyles.NoCurrentDateDefault);
Response.Write(dt.Day + "/" + dt.Month + "/" + dt.Year);
DateTime .ParseExact
DateTime.Parse
To use IFormatProvider you have to pass the culture info for DateTime format coz in different cultures DateTime format of displaying the sequence of month and date varies.
E.g.:
For French Culture use the following:
IFormatProvider culture = new CultureInfo("fr-Fr", true);
For US English use the following:
IFormatProvider culture = new CultureInfo("fr-Fr", true);
Both cultures mentioned above accept the different DateTime Formats.
If you are using
DateTime.Parse
then you have to pass the MM/dd/yyyy hh:mm:ss
Format of DateTime for French culture and dd/MM/yyyy hh:mm:ss for US English culture. You have to check the format for different cultures by changing the position of month and day.
Using DateTime Parse:
dt = DateTime.Parse(myDateTimeString, culture,DateTimeStyles.NoCurrentDateDefault);
Using DateTime ParseExact:
dt = DateTime.ParseExact(myDateTimeString,"MM/dd/yyyy hh:mm:ss", culture, DateTimeStyles.NoCurrentDateDefault);
C# Code to ParseExact the DateTime String:
string myDateTimeString;
myDateTimeString = "19/02/2008 05:44:00";
IFormatProvider culture = new CultureInfo("fr-Fr", true);
dt = DateTime.ParseExact(myDateTimeString,"dd/MM/yyyy hh:mm:ss", culture, DateTimeStyles.NoCurrentDateDefault);
Response.Write(dt.Day + "/" + dt.Month + "/" + dt.Year);
Subscribe to:
Posts (Atom)
Using Authorization with Swagger in ASP.NET Core
Create Solution like below LoginModel.cs using System.ComponentModel.DataAnnotations; namespace UsingAuthorizationWithSwagger.Models { ...
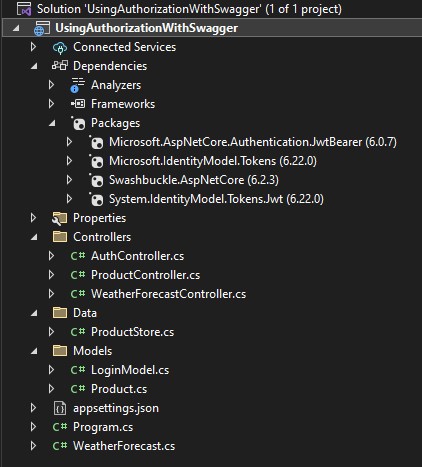
-
<ControlTemplate x:Key="TextBoxBaseControlTemplate" TargetType="{x:Type TextBoxBase}"> <Borde...
-
<?xml version="1.0" encoding="utf-8"?> <Report xmlns:rd="http://schemas.microsoft.com/SQLServer/reporting...
-
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.D...