import javax.comm.*;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.IOException;
public class SerialComm implements SerialPortEventListener
{
CommPort thePort;
SerialPort myPort;
CommPortIdentifier cpi;
InputStream in;
OutputStream out;
String req,index,res1,sql,no;
String res=" ";
String s[]=new String[10];
int z=0;
public SerialComm() throws IOException
{
try
{
cpi = CommPortIdentifier.getPortIdentifier("COM36");
}
catch (NoSuchPortException e)
{
System.out.println("CPI");
}
try
{
thePort=cpi.open("mobile",30000);
myPort=(SerialPort)thePort;
}
catch (PortInUseException e)
{
System.out.println("mobile");
}
try
{
myPort.setSerialPortParams(9600,SerialPort.DATABITS_8,SerialPort.STOPBITS_1,SerialPort.PARITY_NONE);
}
catch (UnsupportedCommOperationException e)
{
System.out.println("SetParam");
}
try
{
in=thePort.getInputStream();
}
catch (Exception e)
{
myPort.close();
System.out.println("InputStream");
}
try
{
out=thePort.getOutputStream();
}
catch (Exception e)
{
myPort.close();
System.out.println("outputStream");
}
try
{
myPort.notifyOnDataAvailable(true);
myPort.notifyOnBreakInterrupt(true);
}
catch (Exception e)
{
System.out.println("DATA avail");
}
try
{
myPort.enableReceiveTimeout(30);
}
catch (UnsupportedCommOperationException e)
{
System.out.println("enable receive");
}
try
{
myPort.addEventListener(this);
}
catch (Exception e)
{
System.out.println("ADD EVENT LISTEN");
}
System.out.println("Opened COM1");
}
public void serialEvent(SerialPortEvent ev)
{
StringBuffer inputBuffer = new StringBuffer();
int newData = 0;
int c=0,dat=0;
char h=0;
switch(ev.getEventType())
{
case SerialPortEvent.DATA_AVAILABLE:
try
{
byte[] b2 = new byte[9];
in.read(b2);
dat = in.read();
dat = dat << 8;
dat += in.read();
closePort();
System.out.println("Closed");
}catch (Exception e)
{
System.out.println("NEWDATA");
System.out.println(e);
return;
}
break;
case SerialPortEvent.BI:System.out.println(" Received Break ");
break;
}
}
class CloseThread extends Thread
{
@Override
public void run()
{
myPort.removeEventListener();
myPort.close();
}
}
public void closePort()
{
try
{
if (myPort != null)
{
myPort.getInputStream().close();
myPort.getOutputStream().close();
new CloseThread().start();
}
}
catch (Exception e) {}
}
}
ASP.NET is a development framework for building web pages and web sites with HTML, CSS, JavaScript and server scripting. ASP.NET supports three different development models: Web Pages, MVC (Model View Controller), and Web Forms.
Subscribe to:
Posts (Atom)
Using Authorization with Swagger in ASP.NET Core
Create Solution like below LoginModel.cs using System.ComponentModel.DataAnnotations; namespace UsingAuthorizationWithSwagger.Models { ...
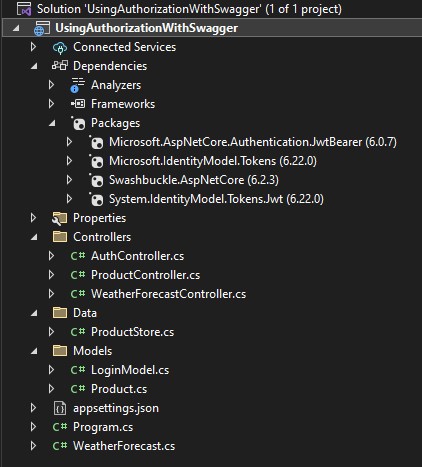
-
<ControlTemplate x:Key="TextBoxBaseControlTemplate" TargetType="{x:Type TextBoxBase}"> <Borde...
-
<?xml version="1.0" encoding="utf-8"?> <Report xmlns:rd="http://schemas.microsoft.com/SQLServer/reporting...
-
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.D...